Behavior-driven development (BDD) is an evolutionary approach to software development that combines business-oriented language with broad team collaboration. Practicing BDD requires a good understanding of business analysis and software development skills.
In this article, we'll be talking about what BDD is, how it's developed, and how it's implemented in the industry.
Introduction to BDD
Behavior-driven development (BDD) is a formal software development process that defines business requirements in terms of features that software should be able to perform. BDD combines business and development operations and makes the two sides codevelop the software in tandem.
First, the teams discuss and agree upon examples of said behavior. Then, they write user stories for them. User stories are general explanations of software features written from the end user's perspective. Through user stories, standard documentation is curated so that all technical and nontechnical teams and stakeholders can understand them.
In BDD, test cases are written in a specific language called Gherkin. They are designed to execute the application's requirements from a user's perspective rather than from an internal programming perspective. Gherkin is a business-readable language. It's not a programming language, and it's not a scripting language. Gherkin is a domain-specific language that is derived from older languages like C.
Advantages of BDD
The greatest advantage of behavior-driven development is that this documentation is achieved in such a manner that it can be developed and tested with automation. The automations are written with the help of test cases that fail till the development has been completed. Thus, the development teams are guided throughout the development of said features.
Another advantage of such documentation, the result of BDD, is that when business teams need to refer to the documentation, they do not need members from the development teams to help them interpret the documentation. This differs from the conventional methods of documentation, such as class diagrams and sequence diagrams. All teams can refer to this one general documentation for reference without it being too technical. It just tells the story in a very simple-to-understand manner.

BDD does not replace your existing adoption of agile methodology but instead enhances it. It encourages working in rapid, small iterations to deliver value frequently. You can take BDD as a set of power-ups for your existing agile process that will enable your team to deliver timely and reliable releases of working software that meets your organization's evolving needs.
How to Implement BDD With a Selenium Framework
There are multiple tools that developers can use to facilitate the process of BDD, and Cucumber is one of them. So, if you are practicing BDD in your organization, you may use Cucumber to automate your documented examples.
Another tool we can throw into this mixture is Selenium, an open-source testing framework used to emulate web browsers to see how applications behave in "real-world" conditions. Cucumber and Selenium pair up to deliver automated BDD implementation, which we'll now take a look at.
Example
We create user stories for any change that we have to implement in the application. It can be a new feature or some existing feature that needs to be upgraded or modified in any manner. As mentioned earlier, these user stories are general explanations of the application feature written from the perspective of the end users. They are often used in agile software development, where teams focus on delivering small, iterative changes to the software.
Let's take an example of a simple authentication system. A user story would be: "As a registered user, I want to access my dashboard." For such a user story, we break the feature down into scenarios using Gherkin, which is a nontechnical language that helps teams describe business behavior without getting into details of implementation. As discussed above, BDD is a collaborative effort. Thus, Gherkin is a framework used to implement BDD that is simple to understand.
Here are the scenarios we have written in Gherkin for the login feature:
We'll place this Gherkin code in a .feature file in ./features/login.feature for Cucumber to pick it up later in this example.
As we can see, Gherkin is just a framework similar to how agile user stories are written: "As a [persona], I [want to], [so that]." It doesn't need to be technical, yet all kinds of technical and nontechnical teams can understand Gherkin scenarios.
Integrating Cucumber With Selenium
Let's install Cucumber and the Selenium web driver package,
Create a file named "cucumber.js" with the following content:
Once we have our Cucumber config and a feature documented in Gherkin, we'll be writing our step definitions in .features/step_definitions/login.js, which will describe the behavior with the help of Selenium.
Once we're done with the step definitions, we're finished. Now we're all set to run our behavior test with npx cucumber-js, which should return the following:
We recommend reading Cucumber's ten-minute tutorial, where multiple advanced scenarios are discussed.
Wrapping Up: A Selenium Framework for BDD
Behavior-driven development is a new paradigm that's rapidly becoming the standard for writing automation tests. This new way of writing tests is custom-tailored for agile development, with many benefits.
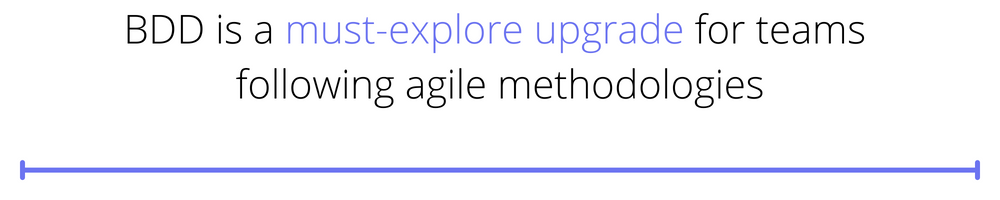
BDD is a must-explore upgrade for teams following agile methodologies, as it upgrades existing processes. Implementing BDD results in quicker development sprints, frequent value delivery, and application behavior documented in a way that even business teams can interpret easily. Cucumber and Selenium are the recommended ways to go for implementing BDD, specifically in a Node.js application with which automated testing can also be achieved in parallel.
If you are a mobile application developer, you need an automated mobile application testing platform like ours to ensure that your app functions correctly and is free of any bugs. Our platform will quickly and easily test your app on various devices, including iPhones, iPads, Android devices, and more. Furthermore, Waldo is constantly updated with the latest mobile operating system versions, so you can be confident that your app will work on the latest devices.
We hope you enjoyed reading about behavior-driven development in Selenium! If you have any questions about this blog post, please let us know in the comments below.
Automated E2E tests for your mobile app
Get true E2E testing in minutes, not months.