Strings are basically collections of characters. And they're similar to what we have in other languages in Swift. In this post, we'll learn to run our Swift code in the Xcode playground.
After that, we'll learn about the basics of strings. Then we're going to look into various examples of how to format Swift strings.

The Setup
We'll run our Swift code in the macOS code editor called Xcode. Since Swift is used for iOS development, the reader is expected to have a Mac machine. We also need to have Xcode installed on our Mac through the App Store.
First, open Xcode and then click on File -> Playground.

On the next screen, select iOS and Blank. And then press the Next button.
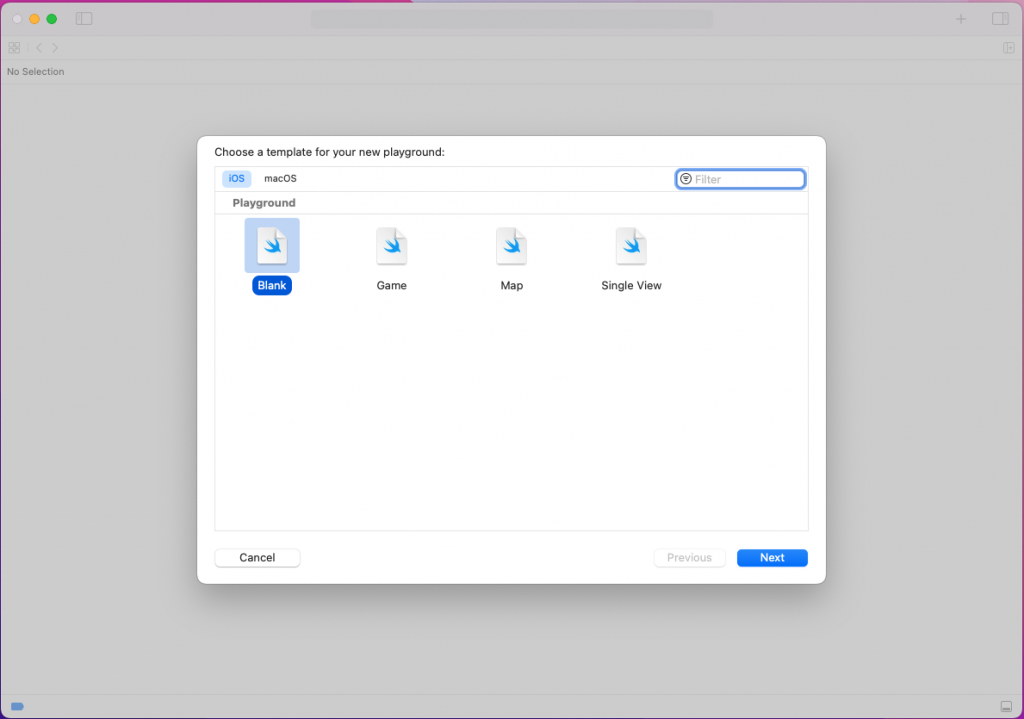
Now we need to give the playground a name. So we'll give it the name StringFormatting. After that, click on the Create button.

Finally, we'll get the playground in which we'll write the code to format the string.

Swift String Basics
We create a string in Swift using a String literal or String class instance. In the playground, we'll create two strings using literal and instance and print them.
Notice that in the first, we're just giving the string inside double quotes. And in the second, we're giving it inside the String() class. Add the below code in the playground:
To run the program and see the result in the console, we need to click on the run button.

Multiline String
We can also write multiple lines of string using triple quote(""") to enclose the string. Here, we'll write the string on multiple lines. And then we'll add the below code in our playground.
Now, we'll be able to see it on our console.

Interpolating String
We can interpolate a string and show constants inside a sentence. In the below example, we've taken three variables, and we're showing them in a sentence.
Notice that to show variables in our sentence, we need to use the \() format.
As shown in the output below, it will interpolate the variables as numbers. And we're also doing an addition operation in it.
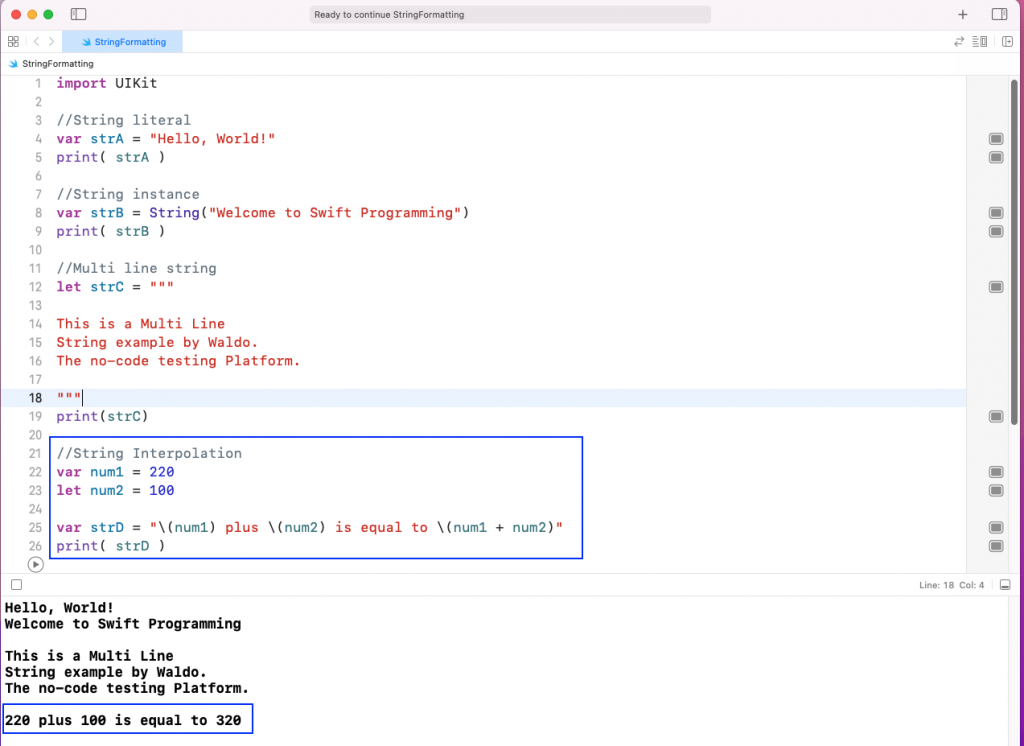
String Functions
We have some useful string functions in Swift, which help us to format the string. We'll look into them in this section.
.png)
Insert Function
We can insert a character in the string using the insert function. But to get the desired index to insert, we need to use the index() function first.
As shown in the below example, we'll get the last index of the string helloStr first. After that, we'll use the insert() function to insert 5 in the last position.
As we can see from the output, 5 is inserted last.

Remove Function
We can remove a character in the string using the remove function. Again, to get the desired index to insert, we need to use the index() function first.
As shown in the below example, we'll get the first index of the string helloStr first. Then, using offsetBy, we'll get the index 8. After that, we'll use the remove() function to remove the element in the eighth position.
We'll also store the removed character in a variable called removed. After that, we'll print both the removed character and the string after removal.
As we can see from the output, character i is removed.
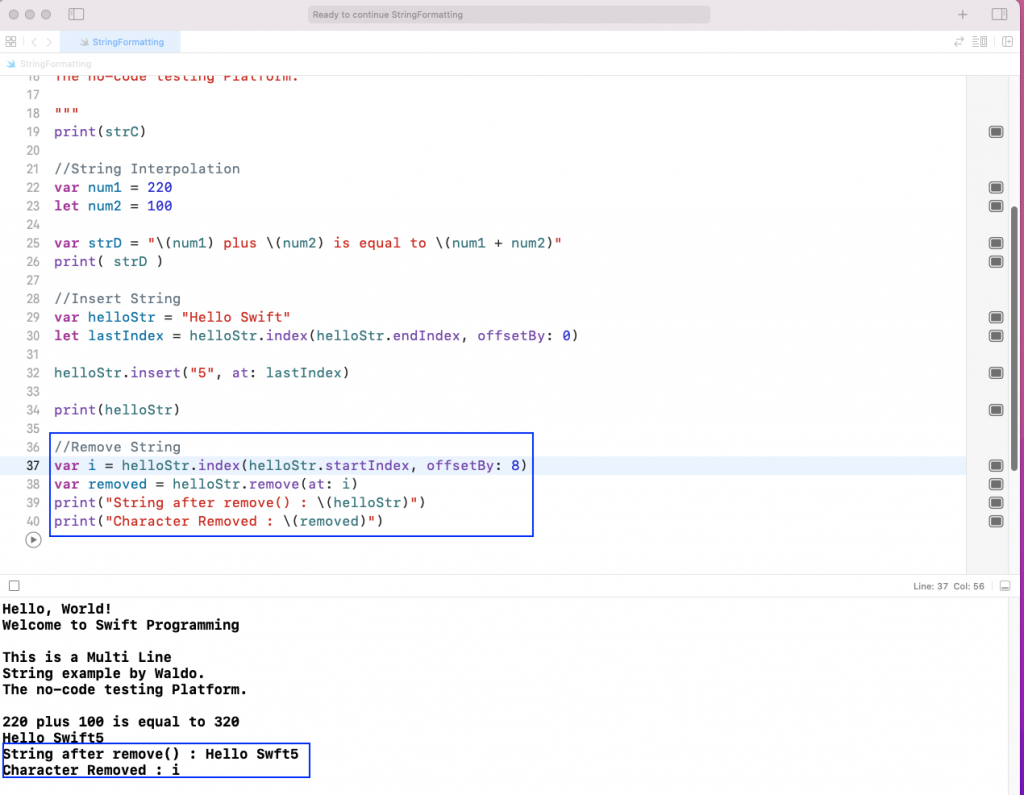
Remove Subrange Function
We can remove more than one character in a string using the remove subrange function.
As shown in the below example, we'll get the first index of the string helloStr first. Then, by using offsetBy, we'll get the index 12. After that, we'll use the removeSubrange() function and give a range in it.
As we can see from the output, Hello Swift was removed.

Reversed Function
We can reverse a string easily with the reversed function. As shown in the below example, we just need to add reserved() to the string to be reversed.
As we can see from the output, Version 5 was reversed. It was reversed to 5 noisreV.

String Concatenation
By string concatenation, we mean the way to add two or more strings. There are three ways to do it in Swift.
Using the Addition Operator (+)
The most common way to concatenate strings is by using the addition operator (+). When we apply this to two strings, it will combine them.
In the below example, we'll combine two strings: nocode1 and nocode2. And we'll store the result in testing.
As shown in the output, the testing string contains the text No code testing with Waldo.

Using the += Operator
We can add a new string to an existing string using the += operator. In the below example, we have a swiftStr variable, and we'll add a new string to it.
As shown in the output, the swiftStr string contains the text Look here for Swift 5.
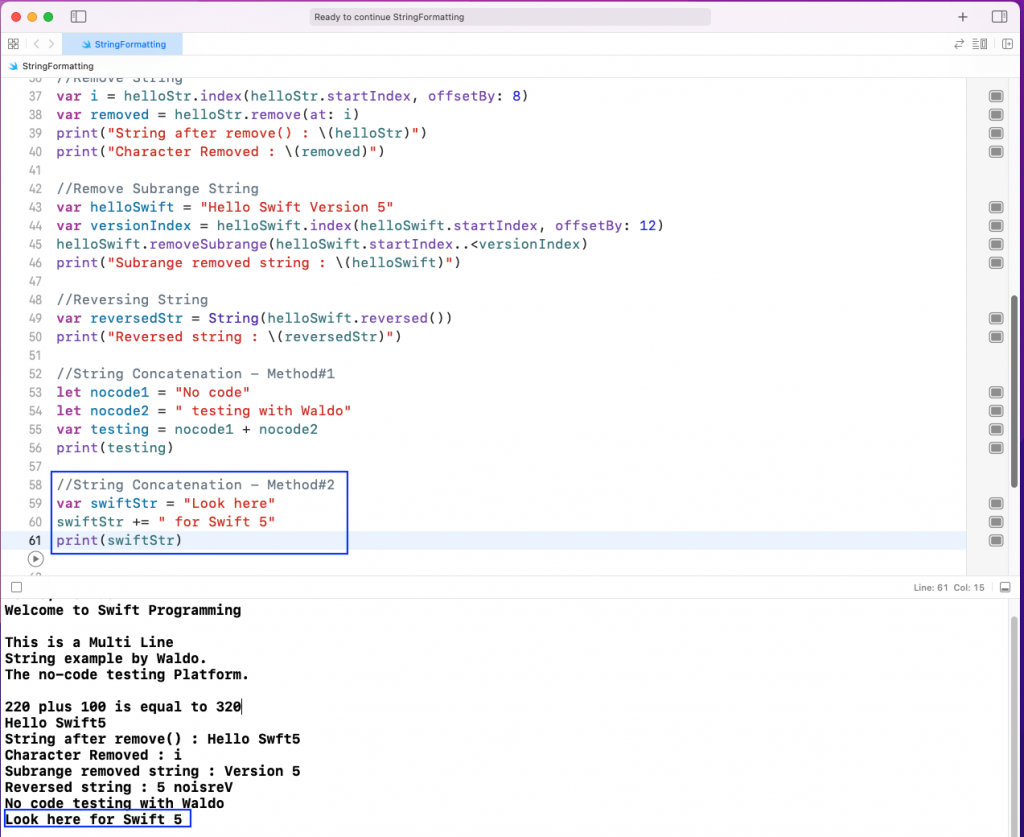
Using the append() Method
We can add a new string to an existing string using the append method. In the below example, we have an iosStr variable. We'll add a new string to it.
As shown in the output, the iosStr string contains the text Look here for iOS 15.4.

Conversion
We can convert a string to an integer. And we can also convert an integer to a string. We'll look into this next.
Converting an Integer to a String
We can convert an integer to a string in the following two ways. Notice that we can do it by putting it inside double quotes. Or we can do it by the String method.
As shown in the output, iosInt is converted into a string.

Converting a String to an Integer
We can convert a string to an integer in the following way.
Notice that a string can also contain characters other than a number.
In the below example, our string is 1982!. So, while converting it, we should always use the optional of ??. And we should give an optional value or else the Swift program could crash.
As shown in the output, we get 2013 because Swift was not able to convert 1982! into a number.
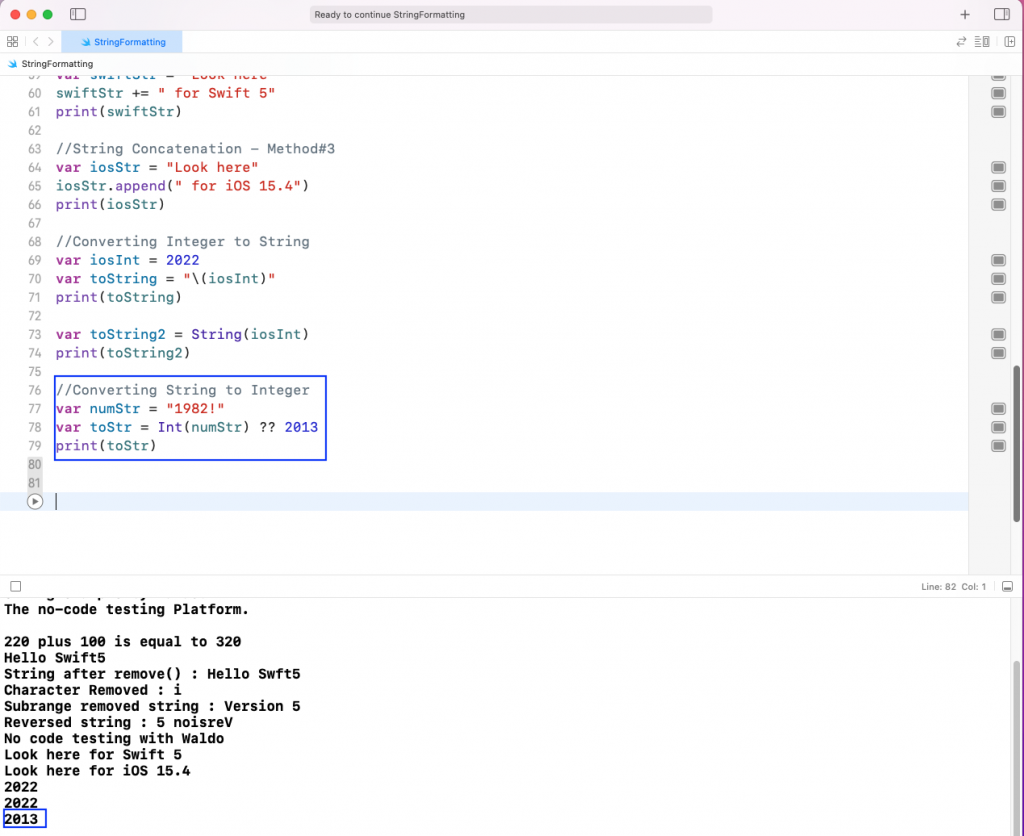
Formatting Strings
We can also change strings to a different format using the String method. It accepts the format parameter in which we can give different types.
In the below example, we'll give the Leading Zero format first with %03d and %05d. Here, 3 and 5 specify the number of zeros including the digit.
Next, we'll specify the number of floating points in a floating point number. We'll use "%.2f" and "%.4f", which specify the number of floating points after the dot.
Lastly, we can also change an integer to hexadecimal format. Here, we need to give "%2X" or "%2x". These specify whether the hexadecimal will be shown in uppercase or lowercase.
We can see in the output the result of all three formattings.
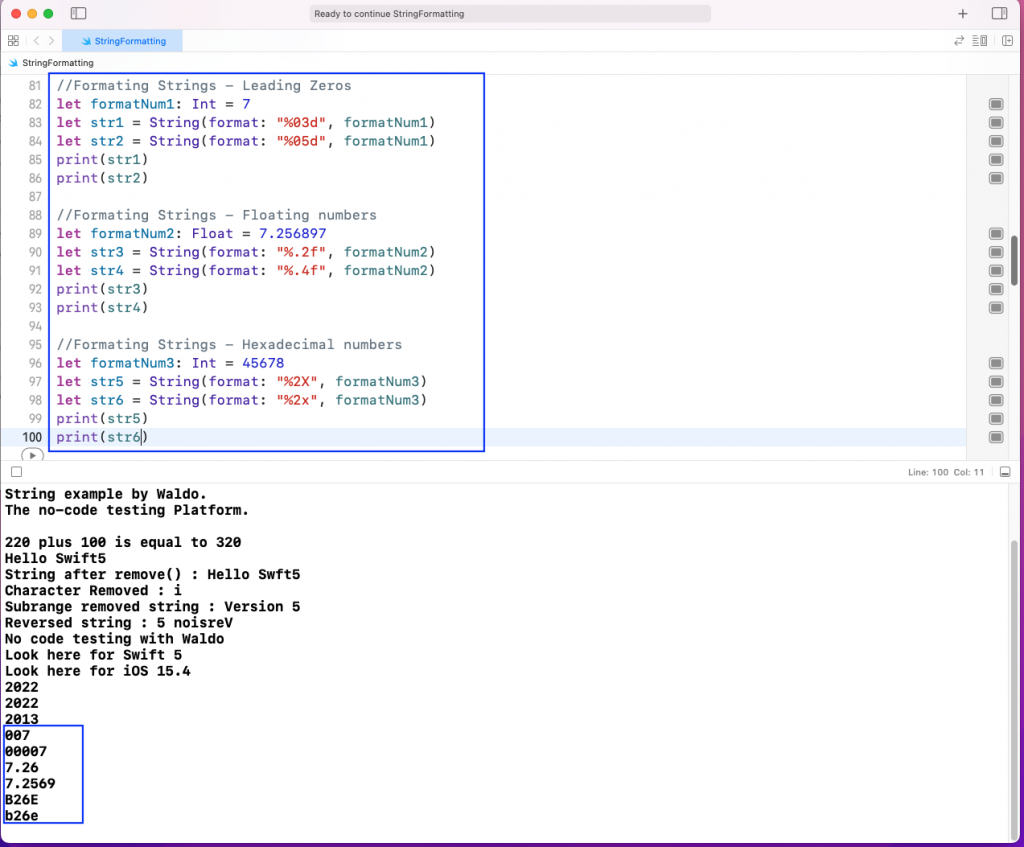
Extension
We can also extend the functionality of string in Swift 5 through Extension. Suppose, in our program, we want the integers to be spelled out. For example, 20 should be written as twenty.
In the below example, we'll use the extension functionality to change the way numbers are interpreted. Using .spellOut is the key here, which changes the number to a string.
On running the code, the 39 will be converted to thirty-nine. But notice that all the other numbers used earlier will also be converted.

Testing With XCTest
We can test our playground code with the XCTest framework. Since we haven't created an iOS app, it will require some more boilerplate code.
First, create a file called TestRunner in the Sources folder and add the below code in it. Here, after doing the required imports, we need to write a structure called TestRunner. Inside it, the code specifies that it will run all test suites.
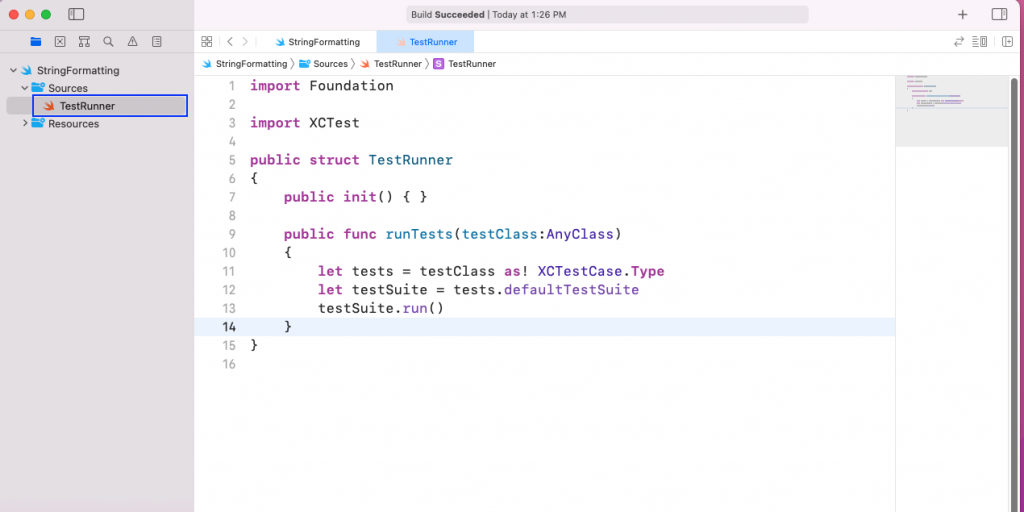
Next, in our playground file called StringFormatting, we'll do the required imports first. After that, we'll write the class StringTests. Inside the class, we'll do an Assert test to check on the string interpolation code.
After that, with TestRunner, we'll run the runTests() function. Here, we'll pass our StringTests.
The code for PlaygroundTestObserver is required to run the test cases in the playground. Lastly, when we run the file, it will show the test case to be successful.

Our only test case ran successfully. However, adding complex things like extensions of strings would require us to write a very complicated test case. Instead, we can use the no-code testing platform Waldo in place of writing all test cases manually.
Waldo can also perform user iteration like clicking on a button. In Waldo, the test cases will be generated automatically, and the test results sent to our inbox.
Create a free Waldo account here and give it a try.
Conclusion
In this post, we've done the basic setup of a playground in which to write our code. After that, we learned about the basics of strings in Swift. Next, we learned the different string functions. After that, we looked at the different string concatenation methods. We checked out the conversion and string format methods. Lastly, we learned to add functionality to strings using the extension method.
Additionally, we tested the app through the XCTest framework, and we learned we can also test the app with the no-code testing platform Waldo.
Automated E2E tests for your mobile app
Get true E2E testing in minutes, not months.