In this article, we'll explore the concept of Flutter enum technology stack. By the end of this article, you'll have a basic understanding of what an enum is, how to implement an enum in Flutter, and how to bind enums to objects.
This article is aimed at Flutter developers and thus requires a basic understanding of Dart and the Flutter technology.
If you haven't explored Flutter and its development stack yet, we recommend you do so here. However, if you're only looking to understand what enums are, you can find more information on our other articles covering your technology of preference.
Let's move on.
What Is an Enum?
Before we dive into the specifics of Flutter enum, let's first explore what enums are.
Enums, or enumerated types, are a data type consisting of a set of named values called elements, members, numerals, or enumerators of the type.
In essence, an enum allows a programmer to use a custom type with a restricted set of values instead of using an integer to represent a set of values.
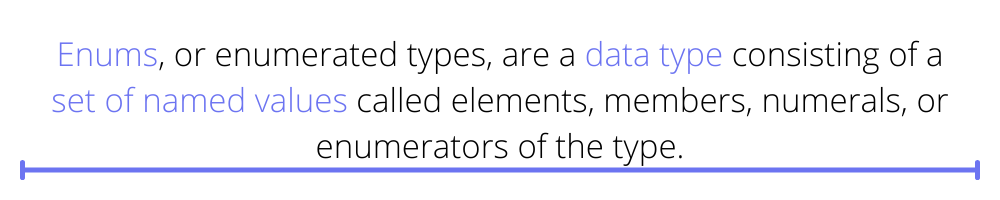
For example, if we use the colors of the rainbow, which are
- red,
- orange,
- yellow,
- green,
- blue,
- indigo, and
- violet,
the compiler will now internally use an int to hold these. If no values are supplied, red will be 0, orange will be 1, etc.
Pretty simple, right?
Great, moving on.
Our First Flutter App
Before we jump into examples of enums in Flutter, we want to build a simple Flutter app that you can use to test the samples that we'll be demonstrating in this article.
In order to create a simple Flutter app, we'll be using the online Flutter compiler DartPad, but if you want to run your apps on your system, feel free to jump to the Flutter guide website here and make sure you have your environment set up. But, again, we'll be keeping it simple for this article and refer only to DartPad.
Now, to create your first Flutter app, all you need to do is make the skeleton for the view and state of the application. Feel free to use this one and add it to DartPad.
If you run this code, you'll be greeted with the following:

Notice that we just have a simple text view with the text "HELLO WORLD!" in it. For the purpose of this article, we'll be restricting our code to this structure, but feel free to play with it and modify whatever you want.
Once you feel like you understand what's going on with the view structure in this example, you can continue to the rest of the article.
Now, let's explore an example of a simple enum in Dart.
Examples of Flutter Enums
As mentioned above, an enum is used to define named constant values.
An enumerated type is declared using the keyword "enum."
Creating a Flutter Enum
Following the colors of the rainbow example, here's an enum representing the same data in Dart:
Notice that we didn't define any integer values.
In Dart, inputting these values is unnecessary since each of the symbols in the enumeration list stands for an integer value.
By default, the value of the first enumeration symbol is 0, which essentially denotes an index of the order of items in the enum.
If you want to see them in action, you can run the following code, see for yourself, and confirm if the result matches our prediction.
Setting Values on a Flutter Enum
It's important to note that enums normally cannot be set to a value after compilation, as they aren't intended to be mutable.
As mentioned by one of the developers of Dart, "As of now, Dart enums are not mutable structures."
Getting Values From a Flutter Enum
However, getting values from an enum—that's another matter entirely.
All you need to do to get the value of an enum in Dart is refer to the specific property or index of the value you want.
Run the code and confirm if it's printing the colors and their index according to the expected result.
Additionally, you can add enums to class objects and use them as properties to define and retrieve values for your object instances.
A simple example would be something like the following:
Notice that you can directly check and compare the value of an enum with the definition itself without needing to refer to its index. This behavior happens because Dart is smart enough to know that each enum is a type of its own and can check them at compile and runtime.
Binding Flutter Enums
Now that we've explored the fundamentals of enums and how to represent them in Dart, let's see how we can do more complex stuff like binding an enum to a view.
For this example, we'll be binding the color enum to a DropdownButton.
If you have a DropdownButton view already set, then go to the instance where it's initialized and change it to the following:
that we set the DropdownButton type argument to be the enum type.
Running this code would give you the following result:
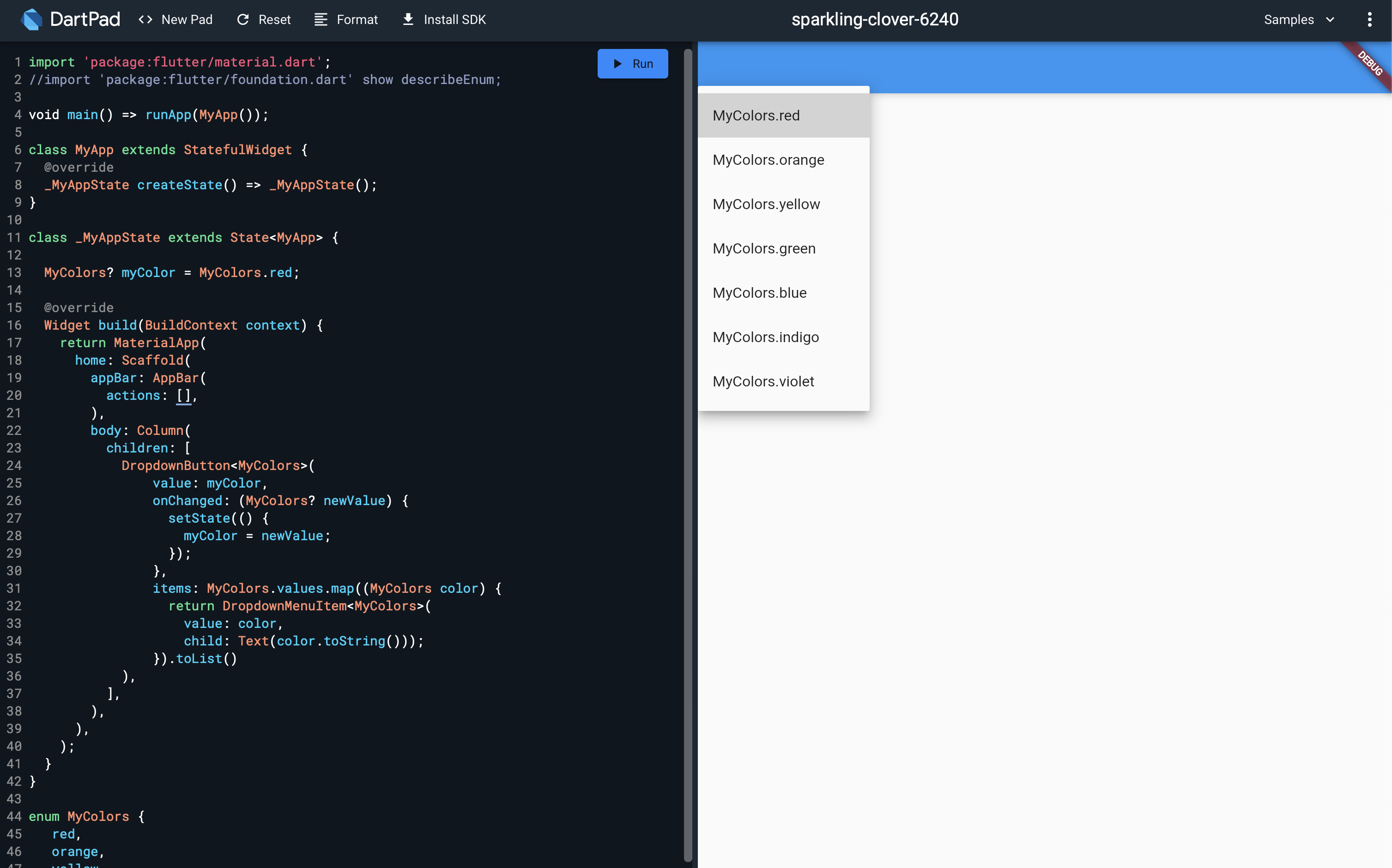
You can now proceed to check the dropdown and see that all the colors are available for you to select.
Extending Flutter Enums
Now, let's say you want to add some extra functionality to your enum. For example, you might want to print some custom text for each value depending on certain conditions, and doing it on each method of your application is not practical.
Well, you can easily do that by extending your enum with extra methods on Dart.
In order to extend our enum, all we need to do is use the "extension" directive and create a special class that contains the methods to add to our enum.
You can see an example of this approach right here:
This code would give you the following result:

Notice that we've added two methods: a displayTitle method that returns the custom string and a color method that returns the actual color object in Flutter.
Quite simple, right?
Alternative
In the process of developing applications, we might face challenges in the form of complex requirements or unforeseen limitations with APIs and hardware. When building complex systems and services with broad reach, it's essential to keep these challenges in mind while optimizing our solution to provide a robust and solid experience for our users.
Sometimes, the cost of development and time to provide that robustness and quality is too great for lean teams with limited experience and resources. In those cases, it's essential to rely on more sophisticated and reliable testing solutions like Waldo's behavioral replay engine.
Our solution can handle your app's dynamic screen, variations in load time, and other common flakiness issues to provide your team with real-time, reliable insights on your product.
You can check it out here.
Conclusion
There's a lot more that can be done to make applications that are very robust and complex. However, for most of the requirements that you might face, this is a good start.
In this post, we've learned what comprises a Flutter application, how to implement enums, and how to make the best use of all the possibilities that the platform offers you. With this knowledge, you should better be able to produce solutions that your clients will love.
Automated E2E tests for your mobile app
Get true E2E testing in minutes, not months.