Writing automated tests is an integral part of the development process as a software developer. It allows us to verify that our code is working as intended and catch any regressions early on.
One of the most common techniques for writing tests in the iOS ecosystem is using Apple's own XCTest framework. With it, you can build simple test cases or extensive test workflows that can help you make sure that your code is robust and reliable.
To achieve this, you can leverage the many assert methods that are available to you right off the bat, like XCTAssertEqual.
In this post, we'll explore XCTAssertEqual in detail so you can bring quality to your work.
First, we'll explain the XCTest framework and how to use it. Then, we'll go into how you can leverage the power of XCTAssertEqual and its sibling methods to ensure that your code is bug-free.
Finally, we'll illustrate all we've learned with a more in-depth example of a test workflow with elaborate test cases and assertions.
If this is your first foray into the world of testing in iOS, we recommend that you also check out this other article on unit testing so you can have a complete understanding of how to work with tests in iOS.
Let's jump right in.
XCTest Basics
XCTest is a testing framework provided by Apple for writing and running unit and performance tests in the iOS, macOS, watchOS, and tvOS ecosystems. It's designed to be easy to use and integrates seamlessly with Xcode, Apple's integrated development environment (IDE).
With XCTest, you can write tests for your code to ensure that it works as intended and catch any regressions early in the development process. To create a test case, you define a subclass of XCTestCase and add one or more test methods. These test methods should have the test prefix and return void.
Here is an example of a simple test case in XCTest:
To run your tests, you can use the XCTest command line tool or select the test target in Xcode and click the "Test" button. In addition, XCTest provides several assertion methods, such as XCTAssertEqual, that you can use to verify the output of your code.

In addition to unit tests, XCTest also provides support for performance tests, which allow you to measure the performance of your code and identify any bottlenecks or optimizations that may be necessary.
You can learn more about these and other tools for testing in this article on swift unit testing.
Working With XCTAssertEqual
Within XCTest, a useful assertion method called XCTAssertEqual allows us to compare two values and determine if they're equal.
Before diving into the specifics of XCTAssertEqual, let's first understand the basics of writing tests in XCTest.
To create a test case, we need to define a subclass of XCTestCase and add one or more test methods. These test methods should have the test prefix and return void.
Once we've defined our test case, we can run it using the XCTest command line tool or by selecting the test target in Xcode and clicking the "Test" button.
Creating a Test Case
Now that we have a basic understanding of how to write tests in XCTest, let's see how we can use XCTAssertEqual to verify that our code is working as intended.
The XCTAssertEqual method takes two arguments: the expected and actual values. If these values are equal, the test will pass. If they're not equal, the test will fail and provide a helpful error message indicating what the expected value and actual value are.
Here's an example of using XCTAssertEqual to test a function that adds two numbers together:
In this example, we call the add function with values 1 and 2 and expect the result to be 3. If the add function returns 3, the test will pass. However, if it returns any other value, the test will fail and provide an error message indicating that the expected value was 3 but the actual value was something else.
One thing to note is that XCTAssertEqual uses the == operator to compare the expected and actual values. This means that it's only suitable for testing values that can be compared using the == operator, such as integers, floats, and strings.
If we want to test for the equality of objects, we need to use a different assertion method, such as XCTAssertEqualObjects.
Customizing Our Assertions
In addition to the expected and actual values, XCTAssertEqual also takes an optional third argument: a message to display if the test fails. You can use this message to provide more context or additional information about the test. For example:
If the test fails, the error message will include the additional message: "The result should be 3."
There are also a few variations of XCTAssertEqual that allow us to specify a tolerance when comparing floating point values. This can be useful when dealing with precision errors or rounding issues. For example, here's an example of using one of the tolerance-based variations of XCTAssertEqual:
This assertion will pass if the actual value is within 0.5 of the expected value (in this case, 3.1). This is great for testing code that performs calculations with floating point values, as these values may sometimes be different due to precision errors.
In addition to XCTAssertEqual, XCTest provides many other assertion methods that can be useful when writing tests. For example, XCTAssertTrue can verify that a boolean value is true, and XCTAssertNil can ascertain that an optional value is nil.
A More In-Depth Example
Here's a more in-depth example of using XCTAssertEqual in an XCTest test case:
In this example, we've defined a test case with four test methods: testAddition, testSubtraction, testMultiplication, and testDivision. Each of these test methods uses XCTAssertEqual to verify that the output of a particular function is what we expect it to be.
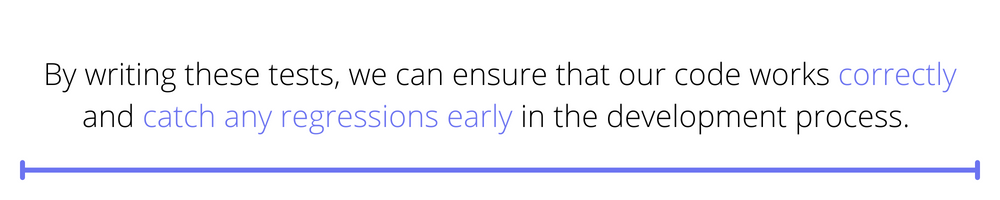
As before, in the testAddition method, we call the add function with the values 1 and 2 and expect the result to be 3. If the add function returns 3, the test will pass. However, if it returns any other value, the test will fail and provide an error message indicating that the expected value was 3 but the actual value was something else.
Similarly, the other test methods use XCTAssertEqual to verify the output of the subtract, multiply, and divide functions.
By writing these tests, we can ensure that our code works correctly and catch any regressions early in the development process.
Conclusion
Overall, XCTAssertEqual is a helpful tool for verifying that the output of our code is what we expect it to be. By writing thorough and well-crafted tests, we can ensure that our code works correctly and catch any regressions early in the development process.
Manual testing may be time-consuming and labor-intensive. This is particularly true for apps with several screens and a relatively complicated user path. The truth is, though, that things don't have to be this way.
You're not alone if you think creating a testing workflow is challenging. The time and effort required to construct all this reasoning is a bad use of many teams' limited resources.
I advise those teams to check out the complete UI testing kit from Waldo.io. Even for non-developers, it's incredibly accessible and doesn't require any code.
Automated E2E tests for your mobile app
Get true E2E testing in minutes, not months.