Strings are collections of characters. They're in all other languages and Swift Strings are quite similar to them. You can learn everything about strings in Swift from our earlier post titled Swift String Formatting Examples.
In this post, we will cover five ways to find string length in Swift. Swift treats Unicode characters like emojis in different ways. And because of that, you need to use a different method to find the length of strings containing Unicode characters.
.png)
Initial Setup
To write the code, we will use XCode, which is the default IDE (Integrated Development Environment) for Mac. We'll write the code in XCode Playground because we are not creating a complete app. Please refer to the Playground Setup from our earlier post titled Exploring Ranges in Swift: Useful Tips and Tricks.
With the setup steps in the post linked above, we created a playground called StringLength.

How Do I Find the Length of a String in Swift?
There are four methods you can use to find the length of a string in Swift.
The Count Method
The most common way to find the length of a string is the count method in Swift. In most other languages, we call it the length method.
In the Swift playground, we have added a string called newStr. We can find its length by newStr.count and assigning it to a variable strLength and then printing it.
We get the output of 28. Note that spaces count as characters.
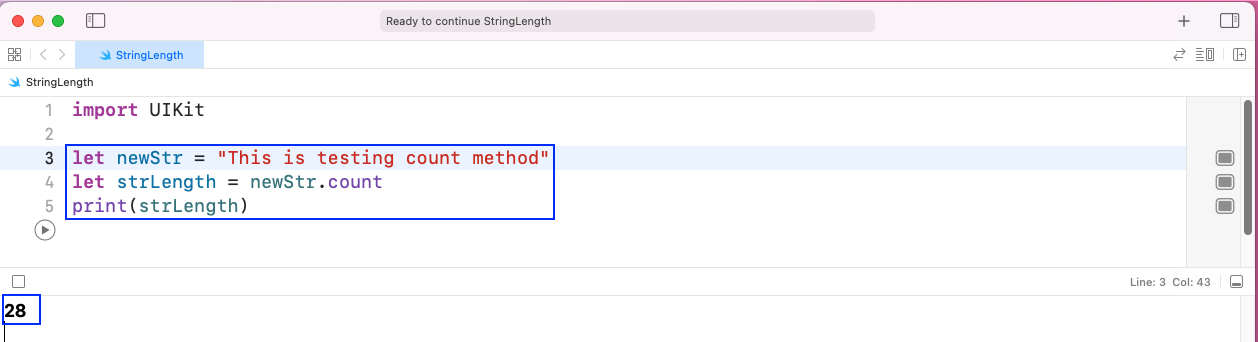
The isEmpty Method
This is technically not a string method as it cannot be used to find the length of a string. But there are cases where we need to know if the string is empty.
Suppose we have a string called latestStr and we want to check whether it's empty. We can do that by using the count method and checking if the string count is zero. In the example below, we have an if statement that will execute only if the string count is zero.
When we run the playground, the statement String is Empty will be displayed indicating that the if statement was run.
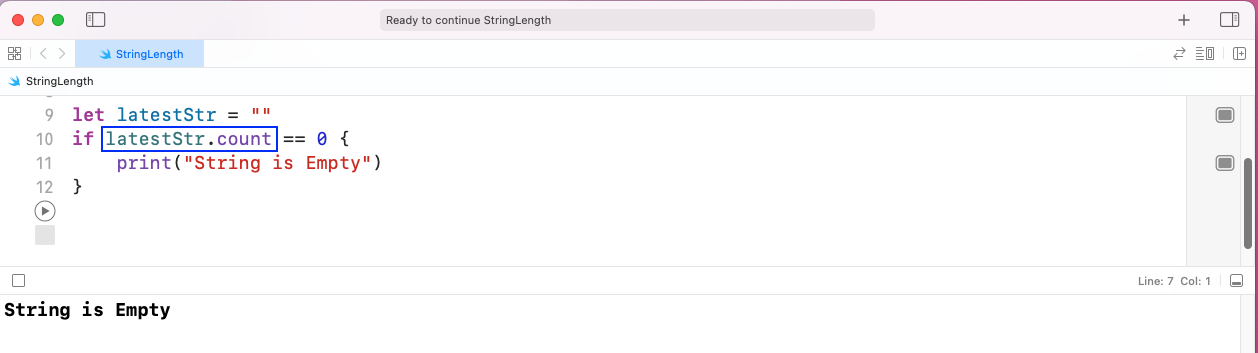
Now, instead of checking the string length with the count method, we can do the same with the isEmpty method. Here, we have updated our if statement with the isEmpty method. The isEmpty method checks if the passed string length is zero.
When we run the playground, the statement String is Empty will be displayed.

Unicode Characters
The next three methods are related to Unicode. You need to learn some basics of Unicode to understand them. All modern computers follow Unicode to store everything. Every character is converted to a number equivalent. For example, the capital A is converted to 65.
There are three different UTF standards: 8-bit UTF-8, 16-bit UTF-16, and 21-bit Unicode Scalar.
Emojis are also Unicode characters. For instance, the emoji 😄 is 128516. When you have emojis in a string, it is advisable to use one of the three methods described next.
The utf8.count Method
The utf8.count method provides the Unicode character length in UTF-8 representation. In the below example, we have a string called emojiStr, which is Swift😄. Notice that it has a smiley face emoji, along with word "Swift." We will use the utf8.count method to get the length of the string emojiStr.
Here, the string length is given as 9 instead of 6 because Emojis have four characters in UTF-8 representation.
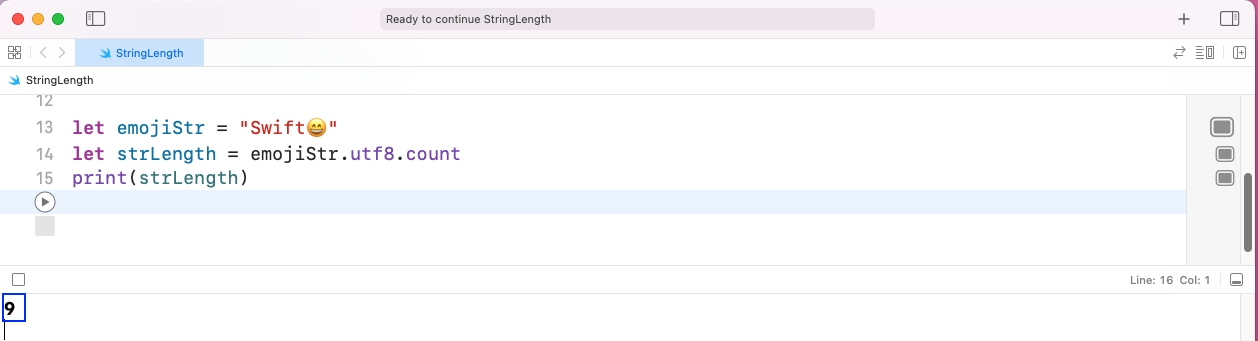
The utf16.count Method
The utf16.count method provides the Unicode character length in UTF-16 representation. In the example below, we have a string called emojiStr, which is Swift😄. We will use the utf16.count method to get the length of the string emojiStr.
Here, the string length is 7 instead of 6 because emojis have two characters in UTF-16 representation.

The unicodeScalars.count Method
The unicodeScalar.count method gives the Unicode character length in Unicode Scalar representation. In the same example, we will use the unicodeScalar.count method to get the length of the string emojiStr.
Here, the string length is 6.

This is the most perfect result as we want an emoji to show one character only. So, if there is no special case, we should use unicodeScalar.count method rather than the other two Unicode methods.
Testing with XCTest
Though you have learned about string length in Playground, it is advisable to test it just like complete mobile apps. In Swift we can test a mobile app or Playground code using the built-in test framework of XCTest. You can learn more in our earlier post titled Swift Switch Statements.
As per the instructions in the post, we need to create a file called "TestRunner" in the Sources folder. This file contains boilerplate code required for testing. Copy the below code in it.

Next, in the StringLength file, we have created a class called StringLengthTests. We have function of testShouldPass() inside it. We have created a variable of emojiStr, which is Swift😄. Now we are using the XCTAssertEqual() to check if the emojiStr.unicodeScalars.count is 6.
The rest of the code is boilerplate required by XCTest. On running the file, we see that the test is successful.

Conclusion
In this post, you learned how to find the length of a string with five different methods. Although in most cases, using the count method works well, in some cases involving emojis, you will need the unicodeScalar.count method.
We also did a bit of testing with XCTest. As you can see above, to write a simple test, you need to write a lot of boilerplate code that you'll need to keep handy. But instead of learning to write all these test cases using all this boilerplate code, you can use Waldo's awesome no-code platform. In Waldo, you only need to upload the APK in the case of Android or the IPA file in the case of iPhone. After that, testers can interact like normal users, clicking and swiping the app. The tool will generate the test cases automatically, and test reports will be available in an email.
To learn more about Waldo's SwifUI support, click here.
Automated E2E tests for your mobile app
Get true E2E testing in minutes, not months.