Swift strings are very powerful, but they can be a little complicated when it comes to indexing into those strings. When you've got a string, how do you get a specific character or substring out of it?
Well, you have a few options. Here are three of the most helpful methods.
What Is an Index in a String?
An index is a number that identifies a character in a string. It's also known as the position of the char in the string.
The index of the first character in a string is zero. A vital thing to note is that the index of the last character in a string will be equal to the length of the string minus one.
Understanding String in Swift
A string is a sequence of characters, and Swift provides a number of ways to work with them. You can create a string by enclosing a sequence of characters in quotation marks:
Once you've created a string, you can perform a number of operations on it, including retrieving individual characters, finding the length of the string, and concatenating strings together.
Swift also provides special support for working with Unicode strings, which can represent a wide variety of languages and symbols. You can use Unicode strings to perform operations such as comparing strings for equality, finding a string's length, and concatenating strings together.
Also Read: Combine for SwiftUi Developers: What It Is and Why You Should Use It
String Index in Swift
Indexing in Swift is a powerful and efficient way to access data stored in an array or dictionary. It enables you to quickly and easily find the data you need without having to search through the entire dataset.
Indexing can be used to access data stored in any order, and it can be used to access data stored in a specific order.
StartIndex and EndIndex in Swift (With Strings)
Swift provides two special methods on its string type that can be used to access substrings: startIndex and endIndex. These methods allow you to specify a range of characters to be extracted from a string.
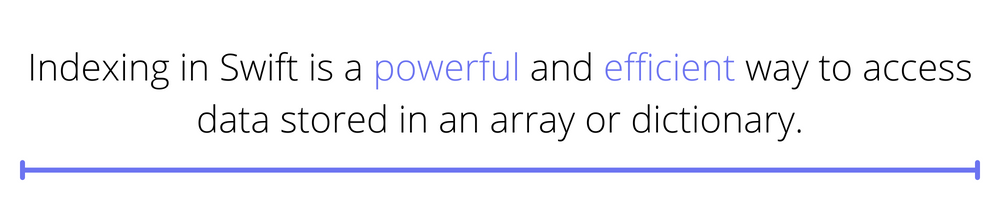
The startIndex is the index of the first character in a string. It's used to specify where a string starts.
Before getting into a more complex example, let's understand a few more concepts.
index(after:) in Swift
The index(after:) method returns the index after the given index. If the given index is the last valid index in the string, this method returns nil.
This method is equivalent to the following code:
Let's understand this with an example:
index(before:) in Swift
The index(before:) method is used to access the index of the element that's located before the given index.
This method is used in conjunction with the index(after:) method to provide access to the elements of a collection in both directions.
For example, the following code extracts the substring "Hello," from a string:
As you can see, using startIndex and endIndex can make it easy to work with substrings in Swift.
Now, let's understand how you can find the index of a substring in a string using those concepts.
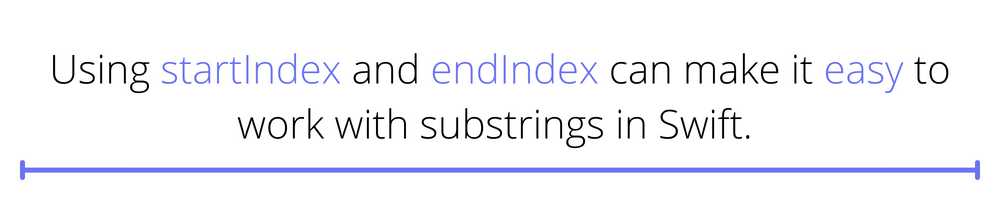
Writing a Function to Find an Index of the Substring (First Occurrence)
When you need to find the index of a substring within a string in Swift, you can use the index(of:) method.
This function returns the index of the first occurrence(first index) of the specified substring or nil if the substring is not found. But in some cases, you might want to create a custom function to return the exact index of a substring in a string.
Finding an Index of the Substring in a String (All Occurrences)
Now, we've written a function to find an index of a substring in a string when that function only returns the first occurrence, but there might be a scenario where we want all occurrences. Let's write a function to accomplish that too.
In the code above, we have a function named findSubstringOccurrences, which takes string and substring as arguments and returns back an array of indexes of the substring in the given string.
Also Read: Swift Struct vs. Class: Here’s How to Decide Which to Use
To Sum Up Swift String Index
In this post, we covered how you can find the index of a substring in a given string, and we've written a function to find indexes of multiple occurrences of a substring in a string. With this knowledge, you'll be able to find a string's index—something that's useful when working with arrays, dictionaries, and other helpful data types in Swift.
If you're a mobile developer and are looking for an automated mobile testing platform, reach out to us. We offer a platform that helps you test your mobile applications automatically so you can focus on developing your app. Our platform is easy to use and enables you to save time and money by automating your mobile testing.
There are many benefits to using such a platform like Waldo, including the ability to automatically test your app on a wide variety of devices and OS versions.
Check out Waldo today to learn more about our automated mobile testing platform. We can help you get started with using the platform and answer any questions you may have.
Automated E2E tests for your mobile app
Get true E2E testing in minutes, not months.